Mocking APIs is a popular practice in software development, with an increasing number of developers now reaping the benefits–and no longer using their valuable time to spin up duplicate resources.
In this article, we explain what mock APIs are, when you should think about using them, and what solutions are available within the open source and proprietary markets. We’ll introduce several different scenarios that demonstrate how mock services are beneficial, so that you can better understand how you might use mock APIs yourself.
What is a mock service?
A mock service is a development tool that imitates a real software service by returning realistic responses–without requiring computed logic to produce the response. For many developers and engineering teams, this capability can be very useful, particularly for cases such as: using third-party APIs that are rate-limited, using an API that isn’t fully developed yet, or when running unit tests or integration tests that include third-party APIs.
Real world mock API example
A good example is Stripe. To process payments for an online storefront, most engineers would utilize the sandbox API that Stripe provides for integration tests. While it’s not the production API, developers can assume that Stripe’s own sandbox API behaves just like it would in production, and can accurately test that everything will work as expected.
Taking this example further, when developers need to figure out how Stripe’s third party API works, and how to form their requests, the sandbox approach is necessary. Further down the development process, however, when they already know how Stripe returns data, they only need to ensure that their application handles the response correctly. This is where mocks come into play.
To create a mock service, you would essentially make a carbon copy of what the third party API returns. Then, you would create a new service that simply responds with the same data on any given endpoint. The mock has no logic at all, and doesn’t call any external services like databases. It only returns static data.
Benefits of mock services
When teams implement mock services within their development process, they tend to see improvements in a few key areas:
Higher quality software
Using mock services can help developers build more robust software by simulating realistic dependencies during the testing process. Developers are able to configure the responses they need, increasing test coverage and quality.
Removing bottlenecks and increasing engineering independence
Engineers no longer need to rely on other engineering resources when they can spin up their own mocks. This helps developers become more independent in their work, as they don’t have to rely on anyone else managing a development or staging environment.
Reducing costs
Financial departments are also a big fan of this approach, as it can greatly reduce resource costs, since the need for a complete staging environment can be eliminated. With mock services, software teams can also bypass fees and/or rate limits imposed by third party providers.
When to use mock services in software testing
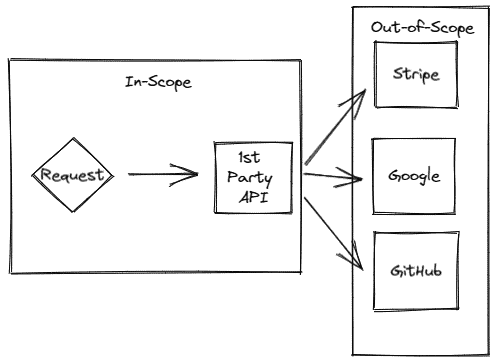
In software testing methodologies, services are defined as being in-scope or out-of-scope. The services that are in-scope are the ones you actually care about when testing. This can be a single service or a cluster of services. Services that are out-of-scope are the ones you don’t want to test, however they may end up being included in your tests if they’re a dependency to an in-scope service.
Mock APIs are most commonly used as part of unit tests, when a service or a cluster of services that have out-of-scope dependencies are being tested. Mock APIs can also be used in integration tests or wherever there is a back-end service that is outside your test boundary. If your in-scope service calls an out-of-scope back-end service, and the out-of-scope back-end service suddenly starts failing, your entire test will fail. This is why it’s so useful to use mock services when testing, as you can be absolutely sure that any out-of-scope services won’t be the reason your test fails. As long as you’re using a proper mocking framework, your mocks will always behave just as you expect them to.
Top use cases for using a mock service
There are many different areas of a developer workflow where mock services can be useful, from the initial building of APIs, to functional testing and performance testing of an application.
Here are some specific examples of how mock APIs can be utilized. We’ve outlined this list so you can understand some of their core use cases and devise new ways to leverage them yourself.
During the initial development of APIs
During the initial development of your APIs, you may be lucky enough that all of your dependencies are third-party services, however, a mock API can still be useful. Mocked services can be a strong tool in cases where there are multiple teams building APIs that depend on each other in some way.
Imagine you’ve just worked out the entire infrastructure plan for a new online store. Team A starts working on the backend for the online store, while Team B is working on the frontend. Team B can start working on the design of the header, footer, and other static things, but how can they start designing the product page? Team A has only just started working on the backend API, so they can’t use the actual backend to get the products. As part of the infrastructure planning, you should’ve made a specification document for the backend API. This means you know exactly what the data from the backend is going to look like, once the API is done. Team B just needs to make sure that the frontend can handle the data.
This is an opportune moment to create a mock API that can return a list of products. Now, Team B doesn’t have to wait for Team A to get the backend finished, they can start working on the entire frontend immediately.
Configuring responses the way you need
When you’re working on an application, you want to make sure that everything works as intended, even if your dependencies aren’t working as expected. It’s very common that you’ll be able to use a sandbox API to test your third-party APIs, which will return perfect sample data. But, do you know how your application will react when you suddenly get a product name that’s five times the length of an average product name? Will the product name wrap to a new line? Will it extend beyond the box? You don’t really have any way of knowing. With a mock API, you can return the exact test data you want.
If you want to have a product name with 255 characters, you can simply configure your mock to return that. Maybe you want to see how your application handles a different, or missing, Content-Type header– you can just change it in your mock. Using a mock API lets you simulate your responses to fit your exact needs.
Testing all possible branches
Much like the previous point, where simulating API responses allows you see how your application will respond to various response modifications, changes to your mock API will also let you test all the different branches of your code. Having an application that doesn’t include at least a few if-statements is fairly rare, and you should always have some form of error handling at a minimum. You may be lucky in that the sandbox API you have access to, also allows you to make requests that intentionally result in errors. Unfortunately, you’re limited to the responses that the API provider created.
With a mocked service, you can define the exact errors you are handling in your application. Most of the time, a frontend application will have generic error-handling, without too much of a distinction between different errors. A backend application, however, will likely have more specific error handling depending on the type of the error. For example, some errors may require a few retries, while others are more serious and require restarting the application. With a mock API, you’re able to replicate all error scenarios and test all branches of your code.
Load testing
While you don’t need mock APIs to do load testing, they can certainly be a big help. Say that you have an application that you want to hit with 50,000 requests, which isn’t unusual. The Service-under-Test may have three dependencies that need to be hit for every request. Suddenly you’re not only load testing your own service, you’re also load testing the back-end service dependencies. The resources required for such a load test can also be expensive. In some cases, you may want to test the resiliency of your entire infrastructure, in which case this is fine, but when you just want to test a single service, you should use a mock API.
Using mock APIs can also help make load testing more reliable. Using mock services allows you to know for certain that all the service dependencies work. Plus, you won’t risk your load tests failing because of a failed dependency.
How Load Testing and Mocks Work Together
Learn how load testing and mocks work together, and
understand the benefits of combining them.
Mocking third party APIs
As mentioned throughout this article, a mock API is a great replacement for a third party API. Using mock APIs allow you to test your application without worrying that you’re hitting any API limits that may have been set by the API provider–a common design within sandbox APIs. To make everything easy, a mock API can be used as a drop-in replacement for your third-party APIs, as long as they follow a conventional protocol like HTTP or gRPC. Of course, this will depend on the tool you’re using to implement your mocks.
Using a mock API as a drop-in replacement is useful when you’re doing local development, but it’s also incredibly useful when it comes to CI/CD, as you can spin up your application and not have to worry about egress rules and API keys. While a sandbox API will likely require an API key (to do rate limiting, for example), a mock API will always return a static response when you hit a specific endpoint–it doesn’t matter what API key you are sending. So for scenarios like this, mock APIs make testing much easier.
Error handling by simulating chaos
Sometimes you know that everything will work as expected; you’ve tested all the paths of your code, and everything seems fine. Although, as most engineers know, nothing is ever perfect. Sometimes something will flip or another service may behave unexpectedly. In general, many different scenarios will happen that you don’t expect. Mock services are great for error handling.
Because mock APIs allow you to configure the exact responses you want, you have the option to introduce chaos, like not returning a response, returning a bad status code, or increasing the response time. This will let you see how your application handles unexpected errors.
Contract testing
An increasingly common methodology in testing is contract testing. With contract testing, you record the interaction between two services and store it in a contract. Then, when you make changes to your application, you need to validate that your application is still upholding that contract. A contract can be something like returning data in a specific schema when a request to your API is made.
In contact testing, when you need to test that your frontend is able to handle the data that it receives from the backend, you don’t actually use the backend. Instead, you pull the contract from a contract store, and then create a mock API based on this. A good tool will be able to help you get this deployed automatically, meaning you can run your tests with a simple command.
How to run a mock service
To run a mock service, you’ll need to decide what data to mock and how to expose this mock service.
Deciding the mock data
Mocking data from real services is challenging because real services oftentimes have dynamic data, or change their interfacing over time. Whenever the real service changes, the mock service must be manually fixed to avoid being obsolete. This makes traditional mock services very brittle, even breaking your test suite.
A new approach to creating mock data called traffic replication has eliminated the brittleness and timelines of traditional mocking. Traffic replication is the process of recording traffic from production to later replay in another environment. Rather than forcing testing teams to create their own mock data, traffic replay allows your real production data to be your mock data. All seen outbound requests and responses are stored to create a mock service that replays the seen responses on demand. If you’re looking to implement traffic replication, Speedscale leads the space.
Exposing the mock service
Mock services typically require dedicated self hosting or manual injection into your environment.
Various open source tools, such as MockServer and GoMock are available if you’re looking to host a dedicated service just for mocking. This will require you to spin up dedicated infrastructure yourself though. If you don’t want dedicated mocking infrastructure, you can also inject mocking into your existing projects, like Mock Service Worker, which injects into your frontend to intercept traffic at the network level.
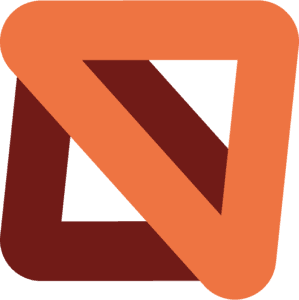
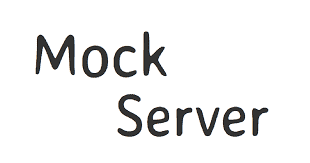
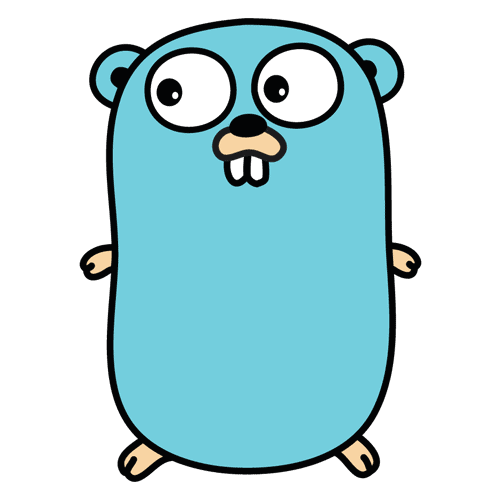
Both of these approaches still succumb to the same brittleness and timelines problems experienced with choosing mock data, since human intervention is still required to set up mock endpoints manually.
Solutions like Speedscale are provisioned and handle service mocking automatically. Speedscale uses traffic replication, so you’ll never manually write brittle mocks because your mocks get automatically created from production traffic. Speedscale also provides advanced features like chaos engineering, PII redaction, load testing, and more, all out of the box.
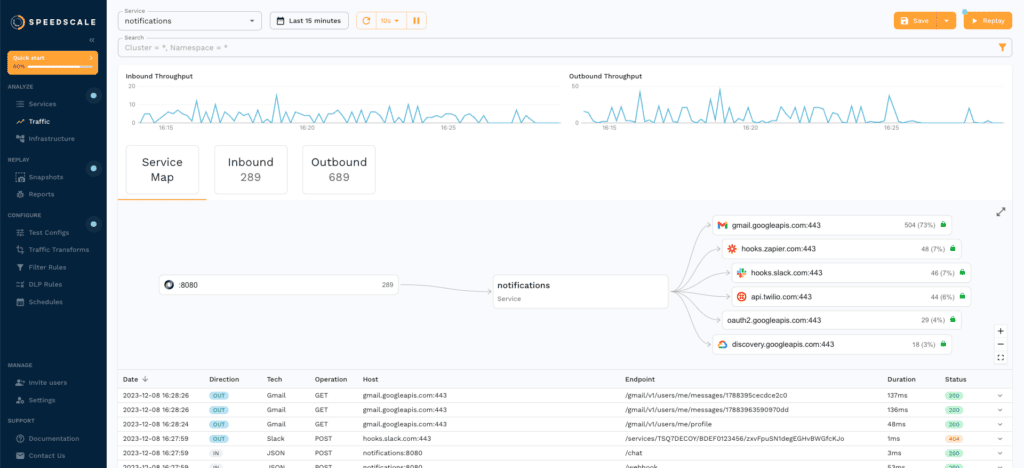
Auto generating mocks with Speedscale
How do you get started mocking services? Check out our post for our top 5 picks for API mocking tools, where we evaluate popular open source and proprietary tools on the market, and how they compare to Speedscale.
API Mocking Tools: Our Top 8 Picks
Learn about the benefits, drawbacks, and key use cases for our top 5 API mocking tools: Postman, MockServer, GoMock, MockAPI, and Speedscale.
If you’re using Kubernetes, we especially encourage you to check out Speedscale. Our production traffic replication platform autogenerates mocks, and allows you to implement mock APIs quickly. Schedule a Demo to see if Speedscale is right for you.