Testing AWS services is an essential step in creating robust cloud applications. However, directly interacting with AWS during testing can be complicated, time-consuming, and expensive. The AWS SDK Mock is a JavaScript library designed to simplify this process by allowing developers to mock AWS SDK methods, making it easier to simulate AWS service interactions in a controlled environment.
Primarily used with AWS SDK v2, AWS SDK Mock integrates with Sinon.js to mock AWS services like S3, SNS, and DynamoDB. This approach helps developers test AWS Lambda functions and other AWS-dependent code without needing actual AWS resources, enhancing test reliability and efficiency.
Throughout this guide, we’ll explore how to set up and effectively use AWS SDK Mock to streamline your AWS testing process.
Setting up AWS SDK Mock is straightforward and can be done quickly to integrate it into your testing environment. Here’s how you can get started:
When using AWS SDK Mock, keep the following in mind:
Mocking AWS services with AWS SDK Mock allows you to test how your application interacts with AWS without needing actual AWS resources. The process begins by using the `AWSMock.mock()` method to simulate specific AWS service methods. For instance, you can mock the `getObject` function of S3 to return a controlled response, such as predefined data or error scenarios, which helps in testing your code under various conditions.
It’s crucial to restore your mocks using `AWSMock.restore()` after each test to avoid unintended interactions between tests. This practice ensures that mocks remain isolated, providing reliable and consistent test results.
AWS SDK Mock also supports custom configurations through `setSDKInstance()`, allowing you to tailor the mocking environment according to your needs, whether you’re working with different SDK versions or unique service setups.
By leveraging these techniques, you can effectively simulate AWS services and create more robust tests that accurately reflect real-world conditions without the complexities and costs of interacting with live AWS environments.
AWS SDK Mock was initially developed to mock AWS Lambda functions, making it especially suited for this use case. It allows developers to simulate AWS service behavior, which is crucial for testing Lambda functions that depend on various AWS services. Although originally designed for Lambda, AWS SDK Mock works effectively with other AWS SDK uses as well.
One important note from the documentation highlights a common issue: when testing Lambda functions, incorrect configurations like missing regions can cause errors such as `ConfigError: Missing region in config`. Proper mocking ensures the SDK behaves as expected.
Using AWS SDK Mock effectively requires understanding best practices and knowing how to troubleshoot common issues. Here are some key tips.
Setting Up AWS SDK Mock
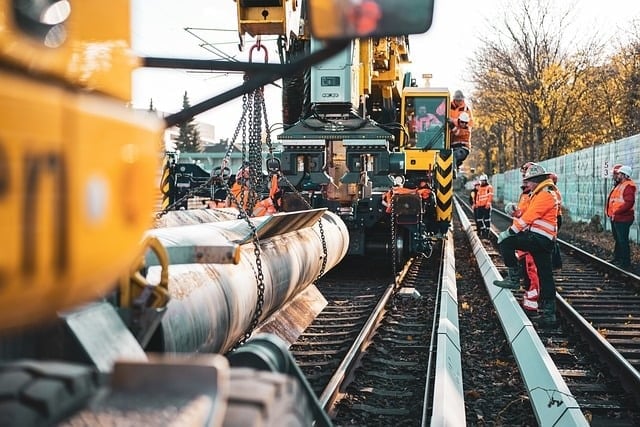
- Install AWS SDK Mock: To install the library, execute the following command using npm:
npm install aws-sdk-mock
- Ensure Compatibility: AWS SDK Mock primarily supports AWS SDK v2. If you’re using AWS SDK v3, consider alternative approaches or ensure v2 compatibility.
- Basic Configuration: Import AWS SDK Mock into your test file and configure it to mock specific AWS services. Here’s an example of how to set it up for mocking S3:
const AWSMock = require('aws-sdk-mock'); AWSMock.mock('S3', 'putObject', (params, callback) => { callback(null, 'success'); });
- Restore Mocks: Always restore AWS SDK Mock between tests to prevent unintended behaviors:
AWSMock.restore('S3');
Key Considerations When Mocking AWS SDK Services
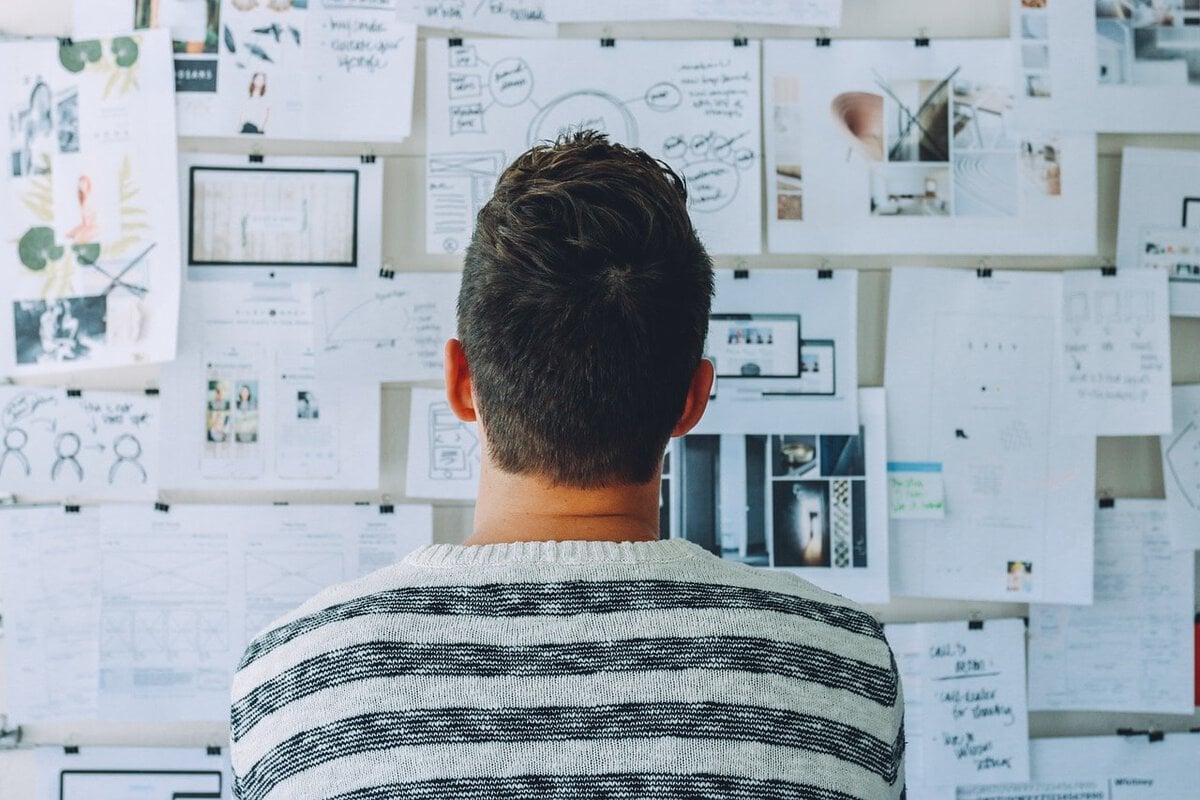
- Version Compatibility: AWS SDK Mock primarily supports AWS SDK v2. Ensure compatibility if your project uses a different version.
- Restoring Mocks: Always use `AWSMock.restore()` to reset mocks after each test to avoid unintended interactions between tests.
- Error Handling: Mocked methods should replicate potential real-world errors to ensure your application can handle failures gracefully.
- Test Isolation: Mocks should be isolated for each test to prevent issues with shared state, thereby enhancing test reliability.
Mocking AWS Services
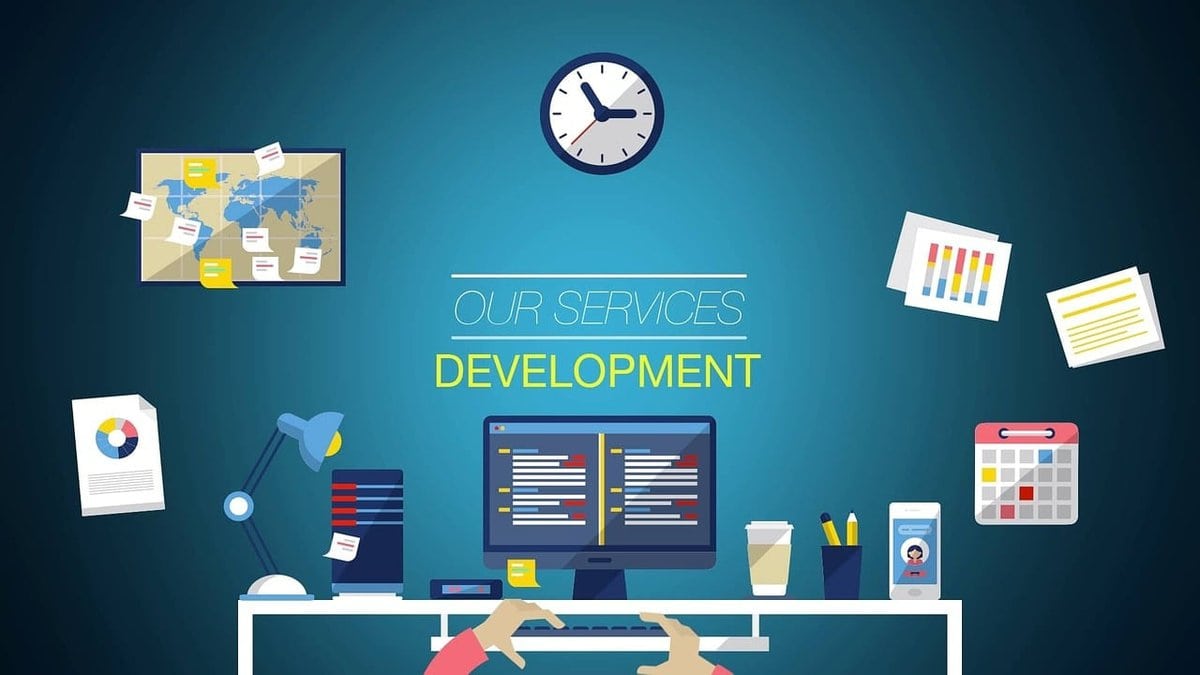
Testing AWS Lambda Functions
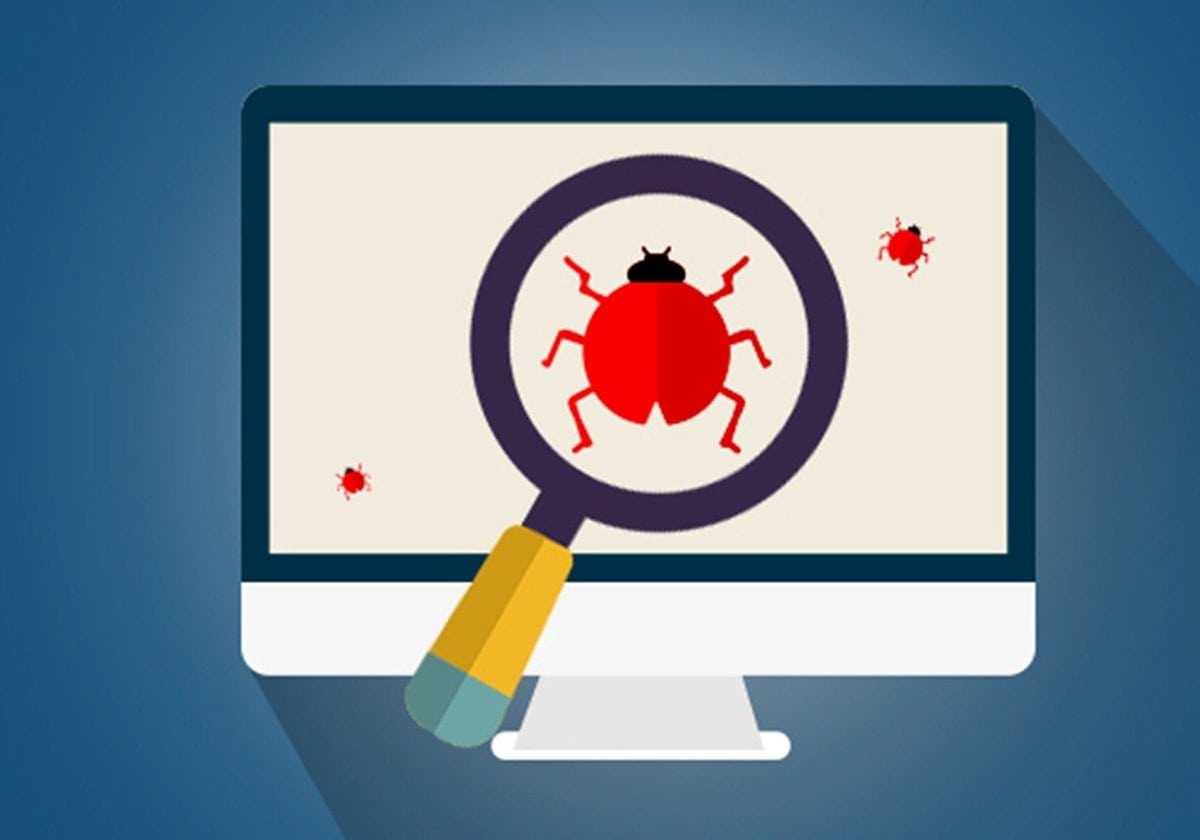
Advanced Usage
AWS SDK Mock offers advanced capabilities beyond basic mocking, allowing developers to tailor their testing environment for more complex scenarios. One of the advanced features is the ability to set custom SDK instances using `setSDKInstance()`. This allows you to mock specific SDK versions or configurations, making the library adaptable to various project needs. Additionally, AWS SDK Mock supports dynamically replacing, restoring, and re-mocking methods, which is especially useful in tests that require different service behaviors. This flexibility enables more comprehensive testing, accommodating a wide range of AWS service interactions. These advanced capabilities make AWS SDK Mock a versatile tool, extending its utility beyond simple mocks and allowing for intricate testing setups that mimic real-world AWS console interactions.Best Practices and Troubleshooting
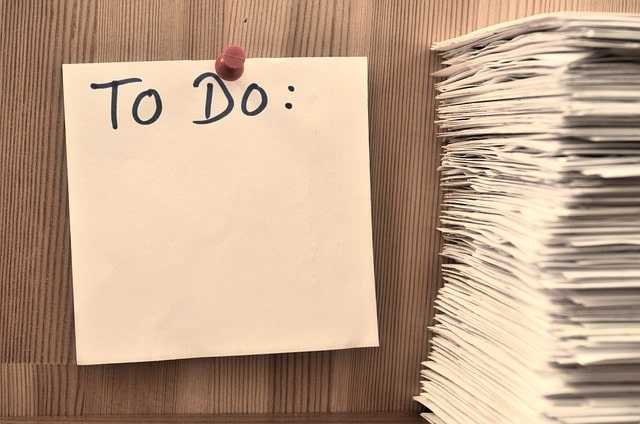